Structured Query Language (SQL) is a set-based language as opposed to a procedural language. It is the defacto language of relational databases.
- Postgresql Psql Commands
- Postgresql Queries Cheat Sheet Pdf
- Postgresql Queries Cheat Sheet Example
- Postgresql Queries Cheat Sheet 2019
- Postgres Json Query Cheat Sheet
- Postgresql Commands Cheat Sheet
- Postgresql Query Example
The PostgreSQL cheat sheet page provides you with the common PostgreSQL commands and statements that enable you to work with PostgreSQL quickly and effectively. Oracle Linux Virtualization Manager (OLVM) Engine PostgreSQL Database Queries Cheat Sheet. This post will explain some practical psql commands and describe how to run the PostgreSQL queries from the command line to get useful information from the engine database.
The difference between a set-based language vs. a procedural language is that in a set-based language you define what set of data you want or want to operate on and the atomic operation to apply to each element of the set. You leave it up to the Database process to decide how best to collect that data and apply your operations. In a procedural language, you basically map out step by step loop by loop how you collect and update that data.
There are two main reasons why SQL is often better to use than procedural code.
- It is often much shorter to write - you can do an update or summary procedure in one line of code that would take you several lines of procedural.
- For set-based problems - SQL is much faster processor-wise and IO wise too because all the underlining looping iteration is delegated to a database server process that does it in a very low level way and uses IO/processor more efficiently and knows the current state of the data - e.g. what other processes are asking for the data.
Example SQL vs. Procedural
If you were to update say a sales person of all customers in a particular region - your procedural way would look something like this
UPDATE customers SET salesperson = 'Mike' WHERE state = 'NH'
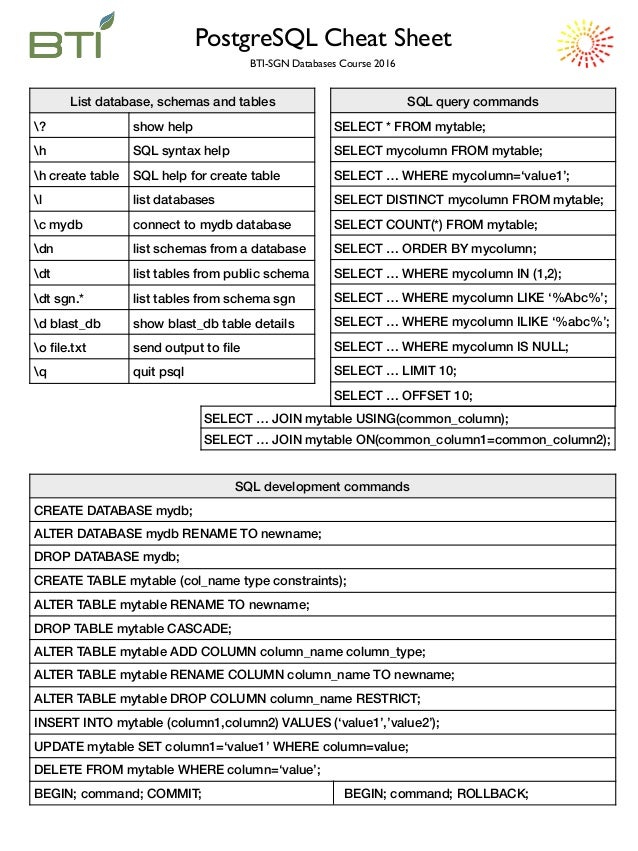
In this article we will provide some common data questions and processes that SQL is well suited for and SQL solutions to these tasks. Most of these examples are fairly standard ANSI-SQL so should work on most relational databases such as IBM DBII, PostGreSQL, MySQL, Microsoft SQL Server, Oracle, Microsoft Access, SQLite with little change. Some examples involving subselects or complex joins or the more complex updates involving 2 or more tables may not work in less advanced relational databases such as MySQL, MSAccess or SQLite. These examples are most useful for people already familiar with SQL. We will not go into any detail about how these work and why they work, but leave it up to the reader as an intellectual exercise. What customers have bought from us?
Example: What customers have never ordered anything from us?
SELECT customers.* FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id WHERE orders.customer_id IS NULL
More advanced example using a complex join: What customers have not ordered anything from us in the year 2004 - this one may not work in some lower relational databases (may have to use an IN clause)
SELECT customers.* FROM customers LEFT JOIN orders ON (customers.customer_id = orders.customer_id AND year(orders.order_date) = 2004) WHERE orders.order_id IS NULL
Please note that year is not an ANSI-SQL function and that many databases do not support it, but have alternative ways of doing the same thing.
- SQL Server, MS Access, MySQL support year().
- PostGreSQL you do date_part('year', orders.order_date)
- SQLite - substr(orders.order_date,1,4) - If you store the date in form YYYY-MM-DD
- Oracle - EXTRACT(YEAR FROM order_date) or to_char(order_date,'YYYY')
Same question with an IN clause
SELECT customers.* FROM customers WHERE customers.customer_id NOT IN(SELECT customer_id FROM orders WHERE year(orders.order_date) = 2004)
How many customers do we have in Massachusetts and California?
SELECT customer_state As state, COUNT(customer_id) As total FROM customers WHERE customer_state IN('MA', 'CA') GROUP BY customer_state
What states do we have more than 5 customers?

Note the above does not work in Microsoft Access or SQLite - they do not support COUNT(DISTINCT ..)
Alternative but slower approach for the above - for databases that don't support COUNT(DISTINCT ..), but support derived tablesList in descending order of orders placed customers that have placed more than 5 orders
Value Insert
Copy data from one table to another table
Creating a new table with a bulk insert from another table
Update from values
UPDATE customers SET customer_salesperson = 'Billy' WHERE customer_state = 'TX'
Update based on information from another table
UPDATE customers SET rating = 'Good' FROM orders WHERE orderdate > '2005-01-01' and orders.customer_id = customers.customer_id
Please note the date format varies depending on the database you are using and what date format you have it set to.
Update based on information from a derived table
Please note the update examples involving additional tables do not work in MySQL, MSAccess, SQLite.
MS Access Specific syntax for doing multi-table UPDATE joins
UPDATE customers INNER JOIN orders ON customers.customer_id = orders.customer_id SET customers.rating = 'Good'
MySQL 5 Specific syntax for doing multi-table UPDATE joins
UPDATE customers, orders SET customers.rating = 'Good' WHERE orders.customer_id = customers.customer_id
Articles of Interest | |
---|---|
PostgreSQL 8.3 Cheat Sheet | Summary of new and old PostgreSQL functions and SQL constructs complete xml query and export, and other new 8.3 features, with examples. |
SQLite | If you are looking for a free and lite fairly SQL-92 compliant relational database, look no further. SQLite has ODBC drivers, PHP 5 already comes with an embedded SQLite driver, there are .NET drivers, freely available GUIs , and this will run on most Oses. All the data is stored in a single .db file so if you have a writeable folder and the drivers, that’s all you need. So when you want something lite and don't want to go thru a database server install as you would have to with MySQL, MSSSQL, Oracle, PostgreSQL, or don't have admin access to your webserver and you don't need database group user permissions infrastructure, this is very useful. It also makes a nice transport mechanism for relational data as the size the db file is pretty much only limited to what your OS will allow for a file (or 2 terabytes which ever is lower). |
PostgreSQL Date Functions | Summary of PostGresql Date functions in 8.0 version |
The Future of SQL by Craig Mullins | Provides a very good definition of what set-based operations are and why SQL is superior for these tasks over procedural, as well as a brief history of the language. |
Summarizing data with SQL (Structured Query Language) | Article that defines all the components of an SQL statement for grouping data. We wrote it a couple of years ago, but it is still very applicable today. |
Procedural Versus Declarative Languages | Provides some useful anlaogies for thinking about the differences between procedural languages and a declarative language such as SQL |
PostgreSQL Cheat Sheet | Cheat sheet for common PostgreSQL tasks such as granting user rights, backing up databases, table maintenance, DDL commands (create, alter etc.), limit queries |
MySQL Cheat Sheet | Covers MySQL datatypes, standard queries, functions |
Comparison of different SQL implementations | This is a great summary of the different offerings of Standard SQL, PostGreSQL, DB2, MSSQL, MySQL, and Oracle. It demonstrates by clear example how each conforms or deviates from the ANSI SQL Standards with join syntax, limit syntaxx, views, inserts, boolean, handling of NULLS |
title: PostgreSQLicon: icon-postgresbackground: bg-blue-800tags:
- nullcategories:
- Databasedate: 2021-01-11 14:19:24intro: |The PostgreSQL cheat sheet provides you with the common PostgreSQL commands and statements.
Getting started {.cols-3}
Getting started
Switch and connect
Postgresql Psql Commands
List all databases
Connect to the database named postgres
Disconnect
psql commands {.col-span-2}
Option | Example | Description |
---|---|---|
[-d] <database> | psql -d mydb | Connecting to database |
-U | psql -U john mydb | Connecting as a specific user |
-h -p | psql -h localhost -p 5432 mydb | Connecting to a host/port |
-U -h -p -d | psql -U admin -h 192.168.1.5 -p 2506 -d mydb | Connect remote PostgreSQL |
-W | psql -W mydb | Force password |
-c | psql -c 'c postgres' -c 'dt' | Execute a SQL query or command |
-H | psql -c 'l+' -H postgres > database.html | Generate HTML report |
-l | psql -l | List all databases |
-f | psql mydb -f file.sql | Execute commands from a file |
-V | psql -V | Print the psql version |
{.show-header} |
Getting help
- | - |
---|---|
h | Help on syntax of SQL commands |
h DELETE | DELETE SQL statement syntax |
? | List of PostgreSQL command |
Run in PostgreSQL console
Working {.cols-3}
Recon
Show version
Show system status
Show environmental variables
List users
Show current user
Show current user's permissions
Show current database
Show all tables in database
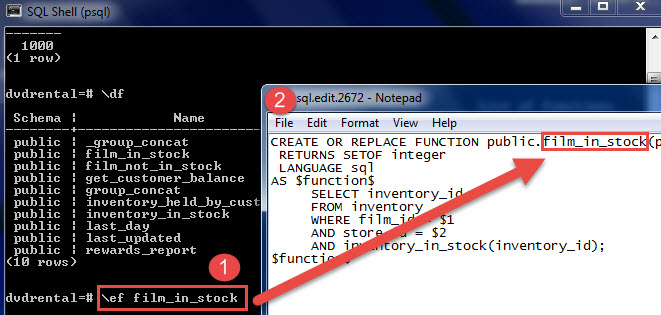
List functions
Databases
List databases
Connect to database
Show current database
Tables
List tables, in current db
List tables, globally
List table schema
Create table, with an auto-incrementing primary key
Permissions
Become the postgres user, if you have permission errors
Grant all permissions on database
Grant connection permissions on database
Grant permissions on schema
Grant permissions to functions
Grant permissions to select, update, insert, delete, on a all tables
Grant permissions, on a table
Grant permissions, to select, on a table
Columns
Update column
Delete column
Update column to be an auto-incrementing primary key
Insert into a table, with an auto-incrementing primary key
Data
[Select](http://www.postgresql.org/docs/current/static/sql-select.html] all data
Read one row of data
Search for data
Insert data
Update data
Delete all data
Delete specific data
Users
List roles
Alter user password
Postgresql Queries Cheat Sheet Pdf
Schema
List schemas
Commands {.cols-3}
Tables
- | - |
---|---|
d <table> | Describe table |
d+ <table> | Describe table with details |
dt | List tables from current schema |
dt *.* | List tables from all schemas |
dt <schema>.* | List tables for a schema |
dp | List table access privileges |
det[+] | List foreign tables |
Query buffer
- | - |
---|---|
e [FILE] | Edit the query buffer (or file) |
ef [FUNC] | Edit function definition |
p | Show the contents |
r | Reset (clear) the query buffer |
s [FILE] | Display history or save it to file |
w FILE | Write query buffer to file |
Informational {.row-span-4}
- | - |
---|---|
l[+] | List all databases |
dn[S+] | List schemas |
di[S+] | List indexes |
du[+] | List roles |
ds[S+] | List sequences |
df[antw][S+] | List functions |
deu[+] | List user mappings |
dv[S+] | List views |
dl | List large objects |
dT[S+] | List data types |
da[S] | List aggregates |
db[+] | List tablespaces |
dc[S+] | List conversions |
dC[+] | List casts |
ddp | List default privileges |
dd[S] | Show object descriptions |
dD[S+] | List domains |
des[+] | List foreign servers |
dew[+] | List foreign-data wrappers |
dF[+] | List text search configurations |
dFd[+] | List text search dictionaries |
dFp[+] | List text search parsers |
dFt[+] | List text search templates |
dL[S+] | List procedural languages |
do[S] | List operators |
dO[S+] | List collations |
drds | List per-database role settings |
dx[+] | List extensions |
S
: show system objects, +
: additional detail
Connection
- | - |
---|---|
c [DBNAME] | Connect to new database |
encoding [ENCODING] | Show or set client encoding |
password [USER] | Change the password |
conninfo | Display information |
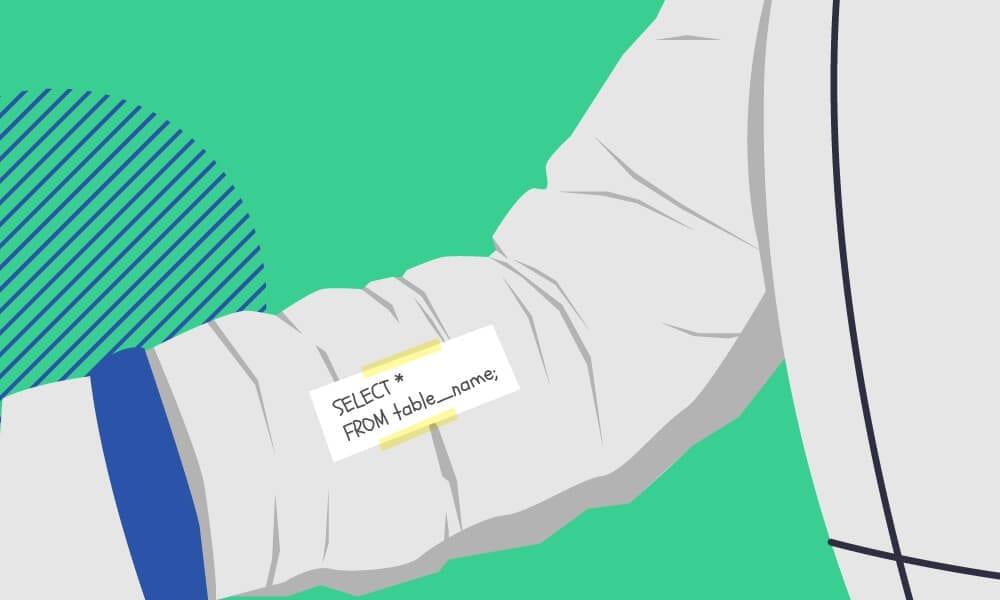
Formatting
- | - |
---|---|
a | Toggle between unaligned and aligned |
C [STRING] | Set table title, or unset if none |
f [STRING] | Show or set field separator for unaligned |
H | Toggle HTML output mode |
`t [on | off]` |
T [STRING] | Set or unset HTML <table> tag attributes |
`x [on | off]` |
Input/Output
- | - |
---|---|
copy ... | Import/export table See also:copy |
echo [STRING] | Print string |
i FILE | Execute file |
o [FILE] | Export all results to file |
qecho [STRING] | String to output stream |
Variables
- | - |
---|---|
prompt [TEXT] NAME | Set variable |
set [NAME [VALUE]] | Set variable (or list all if no parameters) |
unset NAME | Delete variable |
Misc
- | - |
---|---|
cd [DIR] | Change the directory |
`timing [on | off]` |
! [COMMAND] | Execute in shell |
! ls -l | List all in shell |
Large Objects
lo_export LOBOID FILE
lo_import FILE [COMMENT]
lo_list
lo_unlink LOBOID
Miscellaneous {.cols-3}
Backup
Use pg_dumpall to backup all databases
Use pg_dump to backup a database
-a
Dump only the data, not the schema-s
Dump only the schema, no data-c
Drop database before recreating-C
Create database before restoring-t
Dump the named table(s) only-F
Format (c
: custom,d
: directory,t
: tar){.style-none}
Postgresql Queries Cheat Sheet Example
Use pg_dump -?
to get the full list of options
Restore
Restore a database with psql
Postgresql Queries Cheat Sheet 2019
Restore a database with pg_restore
-U
Specify a database user-c
Drop database before recreating-C
Create database before restoring-e
Exit if an error has encountered-F
Format (c
: custom,d
: directory,t
: tar,p
: plain text sql(default)){.style-none}
Use pg_restore -?
to get the full list of options
Remote access
Get location of postgresql.conf
Postgres Json Query Cheat Sheet
Append to postgresql.conf
Append to pg_hba.conf (Same location as postgresql.conf)
Restart PostgreSQL server
Postgresql Commands Cheat Sheet
Import/Export CSV
Export table into CSV file
Import CSV file into table
Postgresql Query Example
See also: Copy
See Also
- Posgres-cheatsheet(gist.github.com)
